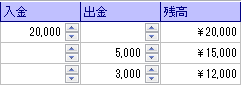
サマリ型セル(SummaryCell)で預金通帳のように入金と出金の履歴に基づく残高を計算するには、ICalculationインタフェースを実装する独自の計算オブジェクトを作成する必要があります。
CustomCalculationクラスのコード
次のコードは、直前の行の残高と入金された値と出金された値の合計を返します。直前の行がない場合(つまり最初の行の場合)は残高を0で処理します。
Imports GrapeCity.Win.MultiRow Public Class CustomCalculation Implements ICalculation Private _balanceCellName As String Private _creditsCellName As String Private _chargesCellName As String Public Function Calculate(ByVal context As GrapeCity.Win.MultiRow.CalculationContext) As Object Implements GrapeCity.Win.MultiRow.ICalculation.Calculate If String.IsNullOrEmpty(Me._creditsCellName) Then Return 0 If String.IsNullOrEmpty(Me._chargesCellName) Then Return 0 If context.Scope = CellScope.Row Then Dim balance As Decimal = 0 Dim credits As Decimal = 0 Dim charges As Decimal = 0 If Not context.SectionIndex = 0 Then Dim balanceCellValue As Object = context.GcMultiRow.GetValue(context.SectionIndex - 1, Me._balanceCellName) balance = DirectCast(balanceCellValue, Decimal) End If Dim creditseCellValue As Object = context.GcMultiRow.GetValue(context.SectionIndex, Me._creditsCellName) If creditseCellValue IsNot Nothing Then credits = DirectCast(creditseCellValue, Decimal) End If Dim chargesCellValue As Object = context.GcMultiRow.GetValue(context.SectionIndex, Me._chargesCellName) If chargesCellValue IsNot Nothing Then charges = DirectCast(chargesCellValue, Decimal) End If Return balance + charges - credits End If Return Nothing End Function ' 残高のセルを指定する Public Property BalanceCellName() As String Get Return _balanceCellName End Get Set(ByVal value As String) _balanceCellName = value End Set End Property ' 出金のセルを指定する Public Property CreditsCellName() As String Get Return _creditsCellName End Get Set(ByVal value As String) _creditsCellName = value End Set End Property ' 入金のセルを指定する Public Property ChargesCellName() As String Get Return _chargesCellName End Get Set(ByVal value As String) _chargesCellName = value End Set End Property Public Function Clone() As Object Implements System.ICloneable.Clone Dim _customCalculation As New CustomCalculation() _customCalculation.BalanceCellName = Me.BalanceCellName _customCalculation.CreditsCellName = Me.CreditsCellName _customCalculation.ChargesCellName = Me.ChargesCellName Return _customCalculation End Function End Class
using GrapeCity.Win.MultiRow; public class CustomCalculation : ICalculation { private string _balanceCellName; private string _creditsCellName; private string _chargesCellName; public object Calculate(CalculationContext context) { if (string.IsNullOrEmpty(this._creditsCellName)) return 0; if (string.IsNullOrEmpty(this._chargesCellName)) return 0; if (context.Scope == CellScope.Row) { decimal balance = 0; decimal credits = 0; decimal charges = 0; if (context.SectionIndex != 0) { object balanceCellValue = context.GcMultiRow.GetValue(context.SectionIndex - 1, this._balanceCellName); balance = (decimal)balanceCellValue; } object creditsCellValue = context.GcMultiRow.GetValue(context.SectionIndex, this._creditsCellName); if (creditsCellValue != null) { credits = (decimal)creditsCellValue; } object chargesCellValue = context.GcMultiRow.GetValue(context.SectionIndex, this._chargesCellName); if (chargesCellValue != null) { charges = (decimal)chargesCellValue; } return balance + charges - credits; } return null; } // 残高のセルを指定する public string BalanceCellName { get { return _balanceCellName; } set { _balanceCellName = value; } } // 出金のセルを指定する public string CreditsCellName { get { return _creditsCellName; } set { _creditsCellName = value; } } // 入金のセルを指定する public string ChargesCellName { get { return _chargesCellName; } set { _chargesCellName = value; } } public object Clone() { CustomCalculation _customCalculation = new CustomCalculation(); _customCalculation.BalanceCellName = this.BalanceCellName; _customCalculation.CreditsCellName = this.CreditsCellName; _customCalculation.ChargesCellName = this.ChargesCellName; return _customCalculation; } }
コーディングによる設定
Imports GrapeCity.Win.MultiRow Dim charges As New NumericUpDownCell() charges.Name = "入金" Dim credits As New NumericUpDownCell() credits.Name = "出金" Dim balance As New SummaryCell() balance.Name = "残高" Dim custom As New CustomCalculation() custom.BalanceCellName = "残高" custom.ChargesCellName = "入金" custom.CreditsCellName = "出金" balance.Calculation = custom balance.Style.TextAlign = MultiRowContentAlignment.MiddleRight Dim Template1 = Template.CreateGridTemplate(New Cell() {charges, credits, balance}) Template1.ColumnHeaders(0).Cells(0).Value = "入金" Template1.ColumnHeaders(0).Cells(1).Value = "出金" Template1.ColumnHeaders(0).Cells(2).Value = "残高" GcMultiRow1.Template = Template1
using GrapeCity.Win.MultiRow; NumericUpDownCell charges = new NumericUpDownCell(); charges.Name = "入金"; NumericUpDownCell credits = new NumericUpDownCell(); credits.Name = "出金"; SummaryCell balance = new SummaryCell(); balance.Name = "残高"; CustomCalculation custom = new CustomCalculation(); custom.BalanceCellName = "残高"; custom.ChargesCellName = "入金"; custom.CreditsCellName = "出金"; balance.Calculation = custom; balance.Style.TextAlign = MultiRowContentAlignment.MiddleRight; Template template1 = Template.CreateGridTemplate(new Cell[] { charges, credits, balance }); template1.ColumnHeaders[0].Cells[0].Value = "入金"; template1.ColumnHeaders[0].Cells[1].Value = "出金"; template1.ColumnHeaders[0].Cells[2].Value = "残高"; gcMultiRow1.Template = template1;